Inheritance in Python
Object-Oriented Programming provides reusable patterns to the code for restricting the redundancy in development projects. One of the basic principles of Object-Oriented Programming that helps achieve recyclable code is Inheritance, where one subclass can leverage the code from another base class.
When one Class utilizes the code constructed with another class is known as Inheritance. We can understand Inheritance by taking a reference from biology; we can take an example as certain traits are inheriting to a child from their parent. These inherited traits can be the eye color or height and even the last name children shared with their parents.
Based on classes’ properties, they are classified into two categories: Parent Class (also known as Super Class or Base Class) and Child Class (also known as Sub Class or Derived Class), respectively.
The Child classes inherit the variables and methods from the Parent Classes. From the above example, we can think of a base class as Parent that has class variables for last_name, eye_color, and height that the derived class Child will inherit from the Parent Class.
Since the subclass Child is inherited from the base class Parent, we can reuse the code of Parent Class in the Child Class. Thus, this principle helps the programmer in reducing the lines of code and decreases redundancy.
- Parent Class: Parent Class is the same as a normal class. It consists of the variables and methods that are mutual and can be reused multiple times. For example, in a college, the student details such as name, date of birth, gender, roll number, course name, admission date are the same in every instance. So, these attributes can be declared in the parent class and used in child classes. Moreover, we can define several functions in the parent, and these functions can be called from multiple child classes.
- Child Class: Child Class looks pretty similar to a normal class. This class inherits all the variables and methods available in its Parent Class. Apart from that, new methods can be created for the child class performing the required calculations. The child class can be constructed in Python by sending the Parent class as an object while constructing a class. This statement implies that a Child class can be created along with parentheses.
A child class can inherit the parent class by mentioning the bracket base after the child class name. Let us consider the following syntax:
Syntax:
1
2
3
4
|
class child_class(parent_class):
<class_suite>
|
Moreover, a Child class can inherit multiple classes by mentioning all the classes inside the bracket. Let us take the following syntax into consideration:
Syntax:
1
2
3
4
|
class child_class(<parent_class_1>, <parent_class_2>, ... <parent_class_n>):
<class_suite>
|
Types of Inheritance in Python
Inheritance is classified into four types depending on the number of parent and child classes involved.:
- Single Inheritance: Inheritance that enables a child class to inherit the attributes and methods from a single parent class is known as Single Inheritance. It enables the reusability of code and add new features to the existing code.

- Multiple Inheritance: Inheritance where a class is derived from more than one parent class is known as Multiple Inheritance. It inherits all the features of the parent classes into the child class.
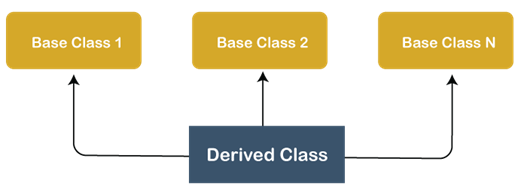
- Multilevel Inheritance: Inheritance where the features of the parent class and the child class are further inherited into the new child class is known as Multilevel Inheritance. Multilevel Inheritance is similar to a relationship that represents a child and grandfather.
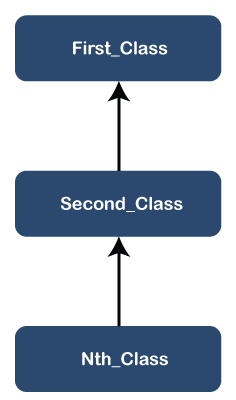
- Hierarchical Inheritance: Inheritance where multiple child classes are derived from a single parent class is known as Hierarchical Inheritance.
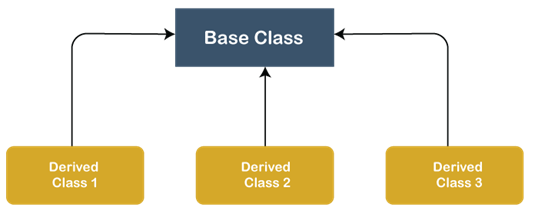
Creating a Parent Class
As we discussed earlier, a Parent Class can be any class. So, the syntax for the Parent Class will remain the same as defining any other class. Let’s consider the following example:
Example:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
class Person:
def __init__(self, f_name, l_name, age, gender):
self.first_name = f_name
self.last_name = l_name
self.age = age
self.gender = gender
def print_details(self):
print(‘Name:’, self.first_name, self.last_name)
print(‘Age:’, self.age)
print(‘Gender:’, self.gender)
info = Person(“Tom”, “Holland”, 15, “Male”)
info.print_details()
|
Output:
1
2
3
4
5
|
Name: Tom Holland
Age: 15
Gender: Male
|
Explanation:
In the above snippet of code, we have created a Person class with some attributes such as f_name, l_name, age, and gender. Then, we have created a print_details() method to print all the details for the attributes provided earlier. We have then added an object info to the Person class.
Creating a Child Class
We can create a child class by passing the parent class as the parameter while creating the class. Consider the following example to understand:
Example:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
|
class Person:
def __init__(self, f_name, l_name, age, gender):
self.first_name = f_name
self.last_name = l_name
self.age = age
self.gender = gender
def print_details(self):
print(‘\nName:’, self.first_name, self.last_name)
print(‘Age:’, self.age)
print(‘Gender:’, self.gender)
info = Person(“Tom”, “Holland”, 15, “Male”)
info.print_details()
class Employee(Person):
pass
info = Employee(“Stacey”, “Cloud”, 23, “Female”)
info.print_details()
|
Output:
1
2
3
4
5
6
7
8
|
Name: Tom Holland
Age: 15
Gender: Male
Name: Stacey Cloud
Age: 23
Gender: Female
|
Explanation –
We have created a Child class Employee under the Parent Class Person and used the pass keyword as we do not want to insert any other attributes or functions to the class.
The __init__() function
Since we have already created a child class that inherits the attributes and properties from its parent class, now, let’s try adding the __init__() function to the child class instead of inserting the pass keyword. It is also known as the constructor.
Note: The __init__() function is automatically called whenever the class is used to create a new object.
Consider the following example demonstrating the insertion of the __init__() function to the Employee class:
Example:
1
2
3
4
5
|
class Employee(Person):
def __init__(self, f_name, l_name, age, gender):
# add some properties etc.
|
Once we insert the __init__() function into the Child class, the parent class’s __init__() function will no longer be inherited by the child class.
Note: The __init__() function of child class overrides the __init__() function’s inheritance of Parent Class.
We can also insert a call to the __init__() function of parent class for keeping the inheritance of the __init__() function of parent class in the child class.
Consider the following example to understand the above statement:
Example:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
|
class Person:
def __init__(self, f_name, l_name, age, gender):
self.first_name = f_name
self.last_name = l_name
self.age = age
self.gender = gender
def print_details(self):
print(‘\nName:’, self.first_name, self.last_name)
print(‘Age:’, self.age)
print(‘Gender:’, self.gender)
info = Person(“Tom”, “Holland”, 15, “Male”)
info.print_details()
class Employee(Person):
def __init__(self, f_name, l_name, age, gender):
Person.__init__(self, f_name, l_name, age, gender)
info = Employee(“Ted”, “Mosby”, 33, “Male”)
info.print_details()
|
Output:
1
2
3
4
5
6
7
8
|
Name: Tom Holland
Age: 15
Gender: Male
Name: Ted Mosby
Age: 33
Gender: Male
|
Explanation –
We have created a Child class Employee under the Parent class Person and define the __init__() function. Then, we have added a class to the __init__() function of the Person class to keep the inheritance of it in the Employee class.
Using the super() function
Python provides a super() function that helps the child class inherit all the attributes and methods from its parent class. We do not have to specify the name of the parent Class while using the super() function as it will inherit the attributes and methods from the parent class automatically. Consider the following example to understand the working of the super() function properly.
Example:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
|
class Person:
def __init__(self, f_name, l_name, age, gender):
self.first_name = f_name
self.last_name = l_name
self.age = age
self.gender = gender
def print_details(self):
print(‘\nName:’, self.first_name, self.last_name)
print(‘Age:’, self.age)
print(‘Gender:’, self.gender)
class Employee(Person):
def __init__(self, f_name, l_name, age, gender):
super().__init__(f_name, l_name, age, gender)
info = Person(“Tom”, “Holland”, 15, “Male”)
info.print_details()
info = Employee(“Daisy”, “Erikson”, 24, “Female”)
info.print_details()
|
Output:
1
2
3
4
5
6
7
8
|
Name: Tom Holland
Age: 15
Gender: Male
Name: Daisy Erikson
Age: 24
Gender: Female
|
Explanation:
In the above snippet of code, we have defined a Parent Class called Person and initialized some attributes in it. We have then created the print_details() method to print all the details provided to it. Later, we have defined a Child Class called Employee and used the super() function to inherit the attributes and methods from the parent class. At last, we have added an object info to the Person Class and the Employee Class, respectively.
Adding Attributes and Methods to the Child Class
The attributes can be added to the Child Class by defining them in the __init__() function of the child class. Similarly, we can add methods to the child class by defining them separately. However, if the method has the same name as the parent class method, the inheritance of the parent’s method will be overridden.
Let’s understand at the following example illustrating the same:
Example:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
|
class Person:
def __init__(self, f_name, l_name, age, gender):
self.first_name = f_name
self.last_name = l_name
self.age = age
self.gender = gender
def print_details(self):
print(‘\nName:’, self.first_name, self.last_name)
print(‘Age:’, self.age)
print(‘Gender:’, self.gender)
info = Person(“Tom”, “Holland”, 15, “Male”)
info.print_details()
class Employee(Person):
def __init__(self, f_name, l_name, age, gender, profession):
super().__init__(f_name, l_name, age, gender)
self.profession = profession
def biodata(self):
print(‘\nFull name: ‘, self.first_name, self.last_name)
print(‘Age: ‘, self.age)
print(‘Gender: ‘, self.gender)
print(‘Profession: ‘, self.profession)
info = Employee(“Mike”, “Tyson”, 43, “Male”, “Wrestler”)
info.biodata()
|
Output:
1
2
3
4
5
6
7
8
9
|
Name: Tom Holland
Age: 15
Gender: Male
Full name: Mike Tyson
Age: 43
Gender: Male
Profession: Wrestler
|
Explanation:
In the above snippet of code, we have created a Parent Class and Child Class as previous one, but added another attribute called profession in the __init__() function of the child Class . We have then created a method inside the Child class to print all the values.
Understanding Multiple Inheritance
The Inheritance in which a Class inherits the properties and methods from more than one base class is known as Multiple Inheritance. This type of Inheritance helps to reduce the redundancy of the Program. However, a certain level of complexity and ambiguity can also be introduced in the Program. Thus, it should be done keeping the overall program design in mind.
The following example illustrates the functioning of Multiple Inheritance:
Example:
1
2
3
4
5
6
7
8
9
10
11
12
13
|
class Fruits:
def f_intro(self):
print(‘Tomato is a Fruit.’)
class Vegetables:
def v_intro(self):
print(‘Tomato is also a Vegetable.’)
class Tomato(Fruits, Vegetables):
pass
name = Tomato()
name.f_intro()
name.v_intro()
|
Output:
1
2
3
4
|
Tomato is a Fruit.
Tomato is also a Vegetable.
|
As we can observe, the child class effectively used methods from both the parent classes.
Multiple Inheritance allows users to utilize the code from multiple parent classes in a child class. If we define the same method in multiple parent methods, the child class will utilize the first parent’s method declared in its tuple list.
Even though Multiple Inheritance can be used effectively, it should be done carefully so that the program does not become ambiguous and complex for other programmers to comprehend.