ARITHMETIC OPERATORS IN COBOL
To go with any operation, where we have to do any calculation or to solve complex equations, we need some arithmetic operators. To do this, COBOL provides us with a set of arithmetic operators to help us in our calculations.
A set of arithmetic operators provided by COBOL is given below:
- add
- subtract
- multiply
- divide
- compute
ADD
This operator is used for addition.
SYNTAX=>
1
2
3
4
5
6
|
ADD (CORROSPONDING/CORR) {identifier 1/literall} TO {identifier 2} GIVING {identifier 3} {ROUNDED}
[ ON SIZE ERROR imperative – statement – 1]
[ NOT ON SIZE ERROR imperative – statement – 2]
END – ADD
|
EXAMPLE=>
1) ADD A TO B
a – 10 , b – 20 , b comes 30
2) ADD CORRESPONDING A TO B.
3) ADD A TO B GIVING C.
a – 10 , b – 20 , then c = 10 + 20 = 30
also
ADD A B GIVING C D
C = A + B
AND D = A + B
4) ADD A TO B ROUNDED.
A – 90.00
B – 10.06
c = 100.06 9(3)V9 THEN 100.1
5) ADD A TO B ROUNDED
ON SIZE ERROR
DISPLAY ” ON SIZE ERROR “.
a – pic 9(2) – 50
b – pic 9(2) – 60
b – 110
6) ADD A TO B ROUNDED
NOT ON SIZE ERROR
DISPLAY ” NOT ON SIZE ERROR “
SAMPLE PROGRAM –
This is a demo program to learn and understand the concept of addition operator.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
|
IDENTIFICATION DIVISION.
PROGRAM–ID. ADDING.
DATA DIVISION.
WORKING_STORAGE SECTION.
01 GP–1.
02 A PIC 9(2) VALUE 50.
02 B PIC 9(2) VALUE 60.
02 C PIC 9(2).
02 D PIC 9(2).
PROCEDURE DIVISION.
MAIN–PARA.
ADD A TO B.
DISPLAY ” THE VALUE OF B BECOMES: “ B.
ADD A TO B ROUNDED
DISPLAY ” THE VALUE OF B NOW IS: “ B.
ADD A TO B ROUNDED
ON SIZE ERROR
DISPLAY ” ON SIZE ERROR: “.
ADD A TO B GIVING C D.
DISPLAY ” THE VALUE OF C IS: “ C.
DISPLAY ” THE VALUE OF D IS: “ D.
STOP RUN.
|
THE OUTPUT WILL BE
1
2
3
4
5
6
7
|
THE VALUE OF B BECOMES: 10
THE VALE OF B NOW IS: 60
ON SIZE ERROR
THE VALUE OF C IS: 10
THE VALUE OF D IS: 10
|

In the above program, we have assigned few variables with different values, and later manipulated the output using different addition operators to clarify the different concepts related to addition operators.
SUBTRACT
This operator is used for SUBTRACTION.
SYNTAX=>
1
2
3
4
5
6
|
SUBTRACT (CORROSPONDING/CORR) {identifier 1/literall} TO {identifier 2} GIVING {identifier 3} {ROUNDED}
[ ON SIZE ERROR imperative – statement – 1]
[ NOT ON SIZE ERROR imperative – statement – 2]
END – SUBTRACT
|
EXAMPLE=>
1) SUBTRACT A FROM B
B = B – A
2) SUBTRACT A FROM B GIVING C.
C = B – A
3) SUBTRACT CORRESPONDING A FROM B.
4) SUBTRACT A B FROM C.
IT IS LIKE C = C – ( A + B )
A = 10
B = 20
C = 50
C = 50 – ( 10 + 20 ) = 20
4) SUBTRACT A TO B ROUNDED.
A – 90.00
B – 10.06
c = 80.06 9(3)V9 THEN 80.1
5) SUBTRACT A TO B ROUNDED
ON SIZE ERROR
DISPLAY ” ON SIZE ERROR “.
6) SUBTRACT A TO B ROUNDED
NOT ON SIZE ERROR
DISPLAY ” NOT ON SIZE ERROR “
SAMPLE PROGRAM –
This is a demo program to learn and understand the concept of subtraction operator.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
|
IDENTIFICATION DIVISION.
PROGRAM–ID. DIFFSUB.
ENVIRONMENT DIVISION.
DATA DIVISION.
WORKING–STORAGE SECTION.
01 GP–1.
02 A PIC 9(2) VALUE 50.
02 B PIC 9(2) VALUE +60.
02 C PIC 9(2).
02 D PIC 9(2).
PROCEDURE DIVISION.
MAIN–PARA.
SUBTRACT A FROM B.
DISPLAY ” THE VALUE OF B BECOMES: “ B.
SUBTRACT A FROM B ROUNDED.
DISPLAY ” THE VALUE OF B IS NOW: “ B.
SUBTRACT A FRIM B ROUNDED
ON SIZE ERROR
DISPLAY ” ON SIZE ERROR “.
SUBTRACT A FROM B GIVING C D.
DISPLAY ” THE VALUE OF C IS: “.
DISPLAY ” THE VALUE OF D IS: “.
STOP RUN.
|
The output will be
1
2
3
4
5
6
|
THE VALUE OF B BECOMES: 1 {
THE VALUE OF B NOW IS: 4 }
THE VALUE OF C IS: 40
THE VALUE OF D IS: 40
|
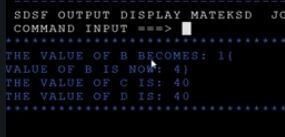
Here in this program, we have assigned few variables with different values and later manipulated the output using different Subtraction operators to clarify the different concepts related to this operator.
MULTIPLY
This operator is used for multiplication of one number to another.
SYNTAX=>
1
2
3
4
5
6
|
MULTIPLY (CORROSPONDING/CORR) {identifier 1/literall} BY {identifier 2} GIVING {identifier 3} {ROUNDED}
[ ON SIZE ERROR imperative – statement – 1]
[ NOT ON SIZE ERROR imperative – statement – 2]
END – MULTIPLY
|
EXAMPLE=>
A) MULTIPLY A BY B
B = A * B
B) MULTIPLY A BY B GIVING C.
C = A * B
C) MULTIPLY A BY B GIVING C D.
C = A * B
D = A * B
SAMPLE PROGRAM –
This is a demo program to learn and understand the concept of multiplication operator and how different types of multiplication operator works.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
|
IDENTIFICATION DIVISION.
PROGRAM–ID. DIFFMUL.
ENVIRONMENT DIVISION.
DATA DIVISION.
WORKING–STORAGE SECTION.
01 GP–1.
02 A PIC 9(2) VALUE 50.
02 B PIC 9(2) VALUE 60.
02 C PIC 9(2).
02 D PIC 9(2).
PROCEDURE DIVISION.
MAIN–PARA.
MULTIPLY A BY B.
DISPLAY ” THE VALUE OF B BECOMES: “ B.
MOVE 60 TO B.
MULTIPLY A BY B ROUNDED.
NOT ON SIZE ERROR
DISPLAY ” NOT ON SIZE ERROR “.
MOVE 10 TOA.
DISPLAY ” A IS NOW: “ A.
DISPLAY ” B IS NOW: “ B.
MULTIPLY A BY B ROUNDED.
ON SIZE ERROR
DISPLAY ” ON SIZE ERROR “.
MOVE 10 TOA.
DISPLAY ” A IS: “ A.
DISPLAY ” B IS: “ B.
MULTIPLY A BY B GIVING C D.
DISPLAY ” THE VALUE OF C IS: “ C.
DISPLAY ” THE VALUE OF D IS: “ D.
STOP RUN.
|
The output will be
1
2
3
4
5
6
7
8
9
10
|
THE VALUE OF B BECOMES: 00
A IS NOW: 50
B IS NOW: 60
ON SIZE ERROR
A IS: 10
BIS: 60
THE VALUE OF C IS: 00
THE VALUE OF D IS: 0600
|
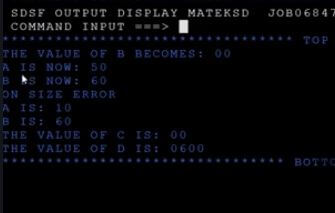
Here in this program, we have assigned few variables with different values and later manipulated the output using different Multiplication operators to clarify the different concepts related to Multiplication operators.
—————————————————————————-
DIVISION
This operator is used for the division of one number by another
SYNTAX=>
1
2
3
4
5
6
7
|
DIVIDE (CORROSPONDING/CORR) {identifier 1/literall} {INTO/ BY} {identifier 2} GIVING {identifier 3}
[ REMAINDER identifier 4 ]
{ROUNDED}
[ { ON SIZE ERROR / NOT ON SIZE ERROR } { imperative – statement } ]
END – DIVIDE
|
EXAMPLE=>
A) DIVIDE A INTO B.
B = B / A
B) DIVIDE A BY B.
A = A / B.
{It is Wrong; never give a command like this, output will show compilation error. }
C) DIVIDE A INTO B BY GIVING C.
C = B / A
D) DIVIDE A BY B GIVING C.
C = A / B
E) DIVIDE A INTO B BY GIVING C D.
C = B / A
D = B / A
F) DIVIDE A BY B GIVING C D.
C = A / B
D = A / B
G) DIVIDE A BY B GIVING C REMAINDER D.
C = A / B
Example= if A = 106 , B = 0
C = 106 / 20 = 5
D = 6
H) DIVIDE A INTO B GIVING C REMAINDER D.
C = B / A
Example= if A = 12 , B = 20
C = 105 / 12 = 8
D = 9
I) DIVIDE A BY B GIVING C REMAINDER D.
ON SIZE ERROR
MOVE 1 TO B.
C = A / B
Example= if A = 106 , B = 0
C = 106 / 0 => anything which is divided by zero is not defined
D = not defined
SAMPLE PROGRAM –
This is a demo program to learn and understand the concept of division operators.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
|
IDENTIFICATION DIVISION.
PROGRAM–ID. DIFFDIV.
ENVIRONMENT DIVISION.
DATA DIVISION.
WORKING–STORAGE SECTION.
01 GP–1.
02 A PIC 9(2) VALUE 50.
02 AA PIC 9(2) VALUE 01.
02 B PIC 9(2) VALUE 60.
02 BB PIC 9(2) VALUE 02.
02 C PIC 9(2).
02 CC PIC 9(2).
02 D PIC 9(2).
02 DD PIC 9(2).
PROCEDURE DIVISION.
MAIN–PARA.
DIVIDE A INTO B.
DISPLAY ” THE VALUE OG B BECOMES: “ B.
MOVE 60 TO B.
DIVIDE A INTO B GIVING AA REMAINDER BB
DISPLAY ” NOT ON SIZE ERROR “
MOVE 00 TOA.
DISPLAY ” A IS MOW: “ A.
DISPLAY ” B IS MOW: “ B.
DISPLAY ” AA IS MOW: “ AA.
DISPLAY ” BB IS MOW: “ BB.
DIVIDE A INTO B ROUNDED
ON SIZE ERROR
DISPLAY ” ON SIZE ERROR “
MOVE 10 TOA.
DISPLAY ” A IS: “ A.
DISPLAY ” B IS: “ B.
MOVE 40 TOA.
MOVE 60 TO B.
DIVIDE A INTO B GIVING C D.
DISPLAY ” THE VALUE OF C IS: “ C.
DISPLAY ” THE VALUE OF D IS: “ D.
DIVIDE A BY B GIVING CC REMAINDER DD.
DISPLAY ” THE VALUE OF CC IS: “ CC.
DISPLAY ” THE VALUE OF DD IS: “ DD.
STOP RUN.
|
The output will be
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
THE VALUE OF B BECOMES: 01
NOT ON SIZE ERROR
A IS NOW: 00
B IS NOW: 60
AA IS NOW: 01
BB IS NOW: 10
ON SIZE ERROR
A IS: 10
BIS: 60
THE VALUE OF C IS: 01
THE VALUE OF D IS: 0001
THE VALUE OF CC IS: 00
THE VALUE OF DD IS: 0040
|

Here in this program, we have assigned few variables with different values and later manipulated the output using different division operators to clarify the different concepts related to division operators.
COMPUTE
COMPUTE is used to assign the value of arithmetic operators present at the right-hand side of ” = ” to the literal/variable that is present at the left-hand side of ” = “. Moreover, compute can combine and solve all the arithmetic operators and assign the result to a single variable.
SYNTAX =>
1
2
3
4
5
|
COMPUTE { Identifier1 / literal1 } [ ROUNDED ]
= { All arithematic operators like + , – , * , / , ** etc }
[ { ON SIZE ERROR / NOT ON SIZE ERROR } { imperative – statement } ]
|
EXAMPLE =>
B = 15 , C =10
1) COMPUTE A = B – C
RESULT , A = 5
2) COMPUTE D ROUNDED = A + B.
RESULT , D = 20
3) COMPUTE E ROUNDED = ( A * B ) / ( D – C )
NOT IN SIZE ERROR
DISPLAY ” NOT ON SIZE ERROR “.
RESULT, E = ( 5 * 15 ) / ( 20 – 10 ) = 75 / 10 = 7
4) COMPUTE F ROUNDED = B * C
ON SIZE ERROR
DISPLAY ” ON SIZE ERROR “.
RESULT, F =15 * 10 = 150
SAMPLE PROGRAM –
This is a demo program to learn and understand the concept of compute operator and how different types of compute operator works.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
|
IDENTIFICATION DIVISION.
PROGRAM–ID. CMTOPR.
ENVIRONMENT DIVISION.
DATA DIVISION.
WORKING–STORAGE SECTION.
01 GP–1.
02 A PIC 9(2).
02 B PIC 9(2).
02 C PIC 9(2).
02 D PIC 9(2).
02 EPIC 9V9.
02 F PIC 9(2).
PROCEDURE DIVISION.
MAIN–PARA.
MOVE 15 TO B.
MOVE 10 TO C.
COMPUTE A = B – C.
DISPLAY ” A IS NOW: “ A.
COMPUTE D ROUNDED = A + B.
DISPLAY ” D IS NOW: “ D.
COMPUTE E ROUNDED = ( A + B ) / ( D – C )
NOT ON SIDE ERROR
DISPLAY ” NOT ON SIZE ERROR “.
COMPUTE F ROUNDED = B * C
ON SIZE ERROR
DISPLAY ” ON SIZE ERROR “.
DISPLAY ” E IS NOW: “ E.
DISPLAY ” F IS NOW: “ F.
STOP RUN.
|
THE OUTPUT WILL BE
1
2
3
4
5
6
7
8
|
A IS NOW: 05
D IS NOW: 20
NOT ON SIZE ERROR
ON SIZE ERROR
E IS NOW: 75
F IS NOW: 00
|

Here in this program, we have assigned few variables with different values and later manipulated the output using different compute operators to clarify the different concepts related to compute operators.